Splitting an String in C++ may seem difficult if you come from a language like Python, Java, or Go, but it’s actually really easy to implement a function that works just like those languages:
#include <string> #include <vector> std::vector<std::string> split(std::string s, std::string delimiter) { if (s.find(delimiter) == std::string::npos) { return std::vector<std::string> {s}; } std::vector<std::string> s_parts {}; size_t pos {0}; std::string token {}; while ((pos = s.find(delimiter)) != std::string::npos) { token = s.substr(0, pos); s_parts.push_back(token); s.erase(0, pos + delimiter.length()); } s_parts.push_back(s.substr(0)); return s_parts; }
As we see, this function takes care of no delimiters in the String, returning just a vector with the original String in it. If the String contains the delimiter, then it will Split it just like it should: returning a vector of all the Strings separated by the chosen delimiter.
You can try this code as follows and see the results for yourself:
#include <iostream> #include <string> #include <vector> std::vector<std::string> split(std::string s, std::string delimiter) { if (s.find(delimiter) == std::string::npos) { return std::vector<std::string> {s}; } std::vector<std::string> s_parts {}; size_t pos {0}; std::string token {}; while ((pos = s.find(delimiter)) != std::string::npos) { token = s.substr(0, pos); s_parts.push_back(token); s.erase(0, pos + delimiter.length()); } s_parts.push_back(s.substr(0)); return s_parts; } void print_vector(std::vector<std::string> vec) { std::string vec_str {}; vec_str += "[ "; for (const auto &s : vec) { vec_str += "\"" + s + "\","; } vec_str.pop_back(); // remove last comma vec_str += " ]"; std::cout << vec_str << std::endl; } int main() { std::string s1 {"How are you doing"}; std::string s2 {"How are you doing "}; std::string s3 {"file"}; std::string s4 {"file.pdf"}; std::string s5 {"file.pdf.OLD"}; std::string s6 {"file.pdf.OLD."}; std::string s7 {".file.pdf.OLD."}; print_vector(split(s1, " ")); print_vector(split(s2, " ")); print_vector(split(s3, ".")); print_vector(split(s4, ".")); print_vector(split(s5, ".")); print_vector(split(s6, ".")); print_vector(split(s7, ".")); }
Note that this functions works with single character strings or with multiple character strings:
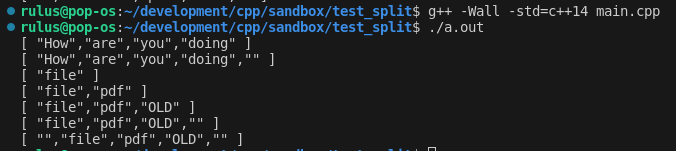